Integration Flow
Learn how to integrate debit card APIs in your application.
The figure below shows the steps needed to create and activate a card.
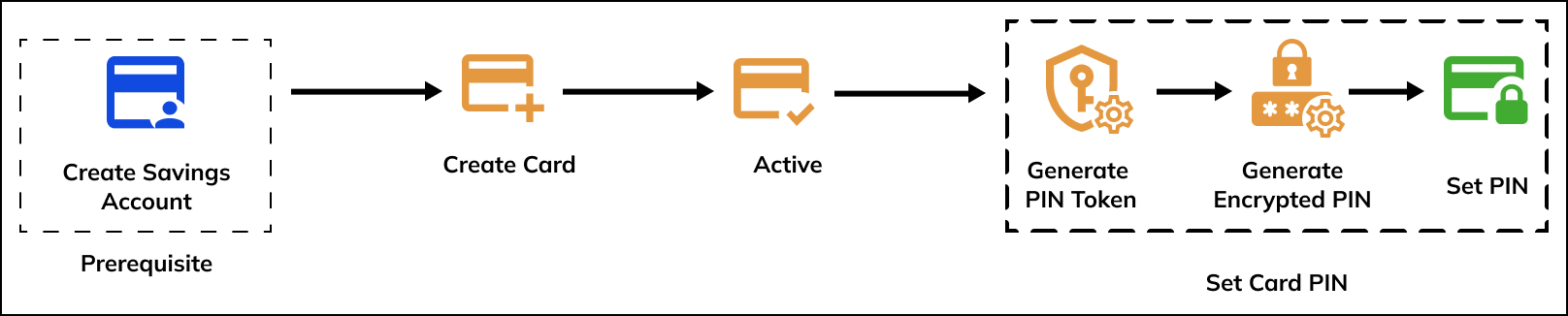
Create Card Workflow
Prerequisite
Currently, debit cards can only be issued against savings accounts. Create a savings account before you create a card.
Follow the below steps to create and activate a card.
1. Create a Debit Card
You can create a debit card using the Create Card API. After the card is successfully created, details are sent to the card manufacturer. The card is manufactured, printed, and shipped to the cardholder.
Use the below endpoint to create a debit card.
POST: https://api.zwitch.io/v1/cards
Below is a sample request and response for the Create Card API.
{
"type": "physical",
"account_id": "sa_aeO72rc0pWy9bubqWEhNRD7SJ"
}
{
"id": "car_R3vZeT6beATdmgD58m1Rcvavf",
"object": "card",
"type": "physical",
"account_id": "sa_aeO72rc0pWy9bubqWEhNRD7SJ",
"kit_id": "00000000001",
"status": "issued",
"cancel_reason": null,
"is_pin_set": false,
"last_4": "0000",
"is_sandbox": true,
"created_at": 1646305675
}
Refer to our API guide for more details about the Create Card API.
2. Activate a Debit Card
After setting a PIN for the card, the card can be activated. The card can only be used after it is activated.
Use the below endpoint to activate a debit card.
POST: https://api.zwitch.io/v1/cards/{card_id}/activate
Below is a sample response for the Activate Card API.
{
"id": "car_R3vZeT6beATdmgD58m1Rcvavf",
"object": "card",
"type": "physical",
"account_id": "sa_aeO72rc0pWy9bubqWEhNRD7SJ",
"kit_id": "00000000001",
"status": "active",
"cancel_reason": null,
"is_pin_set": true,
"last_4": "0000",
"is_sandbox": true,
"created_at": 1646305675
}
Refer to our API guide for more details about the Activate Card API.
3. Set Card PIN
Before a debit card is used for transactions, the cardholder must set a PIN for the card. This is a mandatory step.
Setting the card PIN is a 3-step process.
3.1. Generate Public Key
The first step to setting a PIN for a card is to generate a PIN token. The Generate PIN Token API response contains 2 important parameters, public_key
and id
. These parameters are used to generate the encrypted PIN and set the PIN respectively.
Use the below endpoint to generate a public key.
POST: https://api.zwitch.io/v1/cards/{card_id}/generate-pin-token
Below is a sample response for the Generate Public Key API.
{
"id": "pt_db7ca20c268045f6bcdb27af064c19c4",
"object": "generate_pin_token",
"public_key": "hbv87educbe7889w09i-0qwid90qwfh8wehbfc79we9l",
"card_id": "car_srVIESGzQ4PUCel1xNJg004gb",
"type": "physical",
"account_id": "sa_aeO72rc0pWy9bubqWEhNRD7SJ",
"kit_id": "00000000001",
"card_status": "issued",
"cancel_reason": null,
"is_pin_set": false,
"last_4": "0000",
"is_sandbox": true,
"expires_at": 1641883972,
"created_at": 728728291
}
Refer to our API guide for more details about the Generate PIN Token API.
3.2. Generate Encrypted PIN
This step has to be done on your server.
After generating the PIN token, use the below values to generate the encrypted PIN.
- SHA256 algorithm.
- Values received against the
public_key
parameters in the Generate PIN Token API response. - PIN entered by the cardholder.
SHA256 for Encryption
Use SHA256 to generate the encrypted PIN. Using any other encryption method will lead to errors.
Use the below sample code to generate the encrypted PIN.
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
from Crypto.Hash import SHA256
import base64
def generate_encrypted_pin(public_key, pin):
public_key = base64.b64decode(public_key)
key = RSA.importKey(public_key)
encryptor = PKCS1_OAEP.new(key, SHA256)
encrypted_pin = encryptor.encrypt(pin.encode('utf-8'))
return base64.b64encode(encrypted_pin)
encryptedPin = generate_encrypted_pin("public_key", "pin")
print(encryptedPin)
const forge = require('node-forge');
let key = "<public_key>";
let pin = "<pin>";
const { pki } = forge;
const decodePublicKey = forge.util.decode64(key);
const publicKey = pki.publicKeyFromPem(decodePublicKey);
const encrypted = publicKey.encrypt(pin, 'RSA-OAEP', {
md: forge.md.sha256.create(),
});
const encryptedPin = forge.util.encode64(encrypted);
console.log(encryptedPin);
[Python Only] Install PIP3 Crypto Library
Ensure you have the Python Crypto library installed on your system before using the above python script.
Use the below code snippet to install the Python Crypto library.
pip3 install crypto
3.3. Set PIN
After generating the encrypted PIN, use the Set PIN API to set the PIN for the card. This PIN is required to carry out transactions such as ATM and POS transactions.
Use the below endpoint to set the PIN for a card.
POST: https://api.zwitch.io/v1/cards/{card_id}/set-pin
Below is a sample request and response for the Set PIN API.
{
"pin_token_id": "pt_db7ca20c268045f6bcdb27af064c19c4",
"pin": "kad667igk2=ask-=b7ca20c268045f6bcdb27af064c19c4"
}
{
"id": "car_R3vZeT6beATdmgD58m1Rcvavf",
"object": "card",
"type": "physical",
"account_id": "sa_aeO72rc0pWy9bubqWEhNRD7SJ",
"kit_id": "00000000001",
"status": "issued",
"cancel_reason": null,
"is_pin_set": false,
"last_4": "0000",
"is_sandbox": true,
"created_at": 1646305675
}
The table below lists the request parameters for the Set PIN API.
Parameter | Type | Description |
---|---|---|
pin_token_id | string | Pass the value received against the id parameter in the Generate PIN Token API response here.Example: pt_db7ca20c268045f6bcdb27af064c19c4 |
pin | string | Pass the encrypted PIN generated in the previous here. Example: kad667igk2=ask-=b7ca20c268045f6bcdb27af064c19c4 |
Refer to our API guide for more details about the Set PIN API.
Updated over 1 year ago